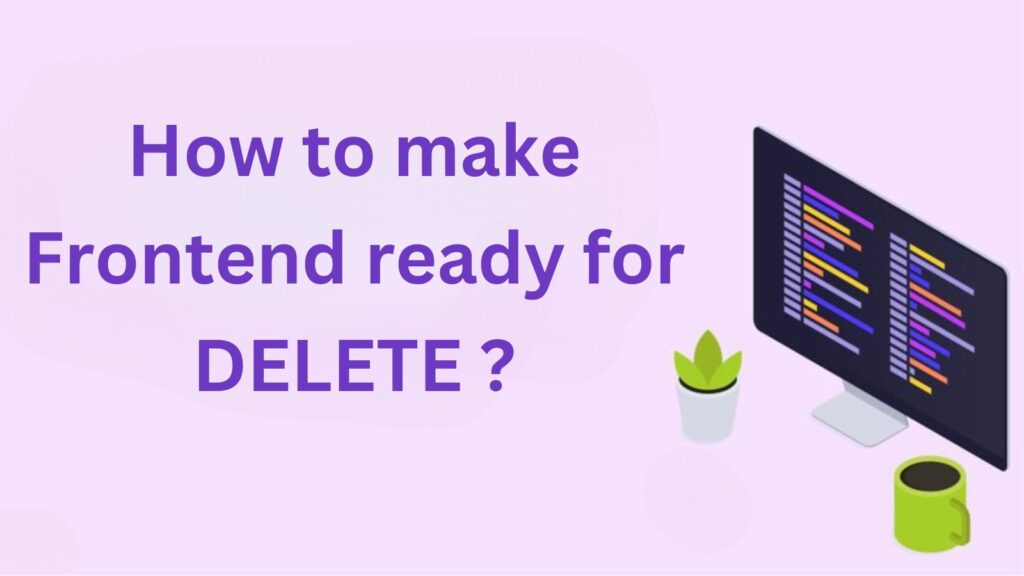
Setup the NextJS Application
Install everything
npx create-next-app@latest my-next-app
npm install axios
Create the UI ( Form ) + Axios DELETE Query
import { useEffect, useState } from 'react'
import axios from 'axios'
export default function Products() {
const [products, setProducts] = useState([])
const [loading, setLoading] = useState(true)
const [name, setName] = useState('')
const [price, setPrice] = useState('')
useEffect(() => {
fetchProducts();
}, []);
const fetchProducts = async () => {
try {
const response = await axios.get('http://localhost:5000/products');
setProducts(response.data);
setLoading(false);
} catch (error) {
console.error('Error fetching products:', error);
}
};
const handleSubmit = async (e) => {
e.preventDefault(); // Prevent page reload
try {
const newProduct = { name, price };
const response = await axios.post('http://localhost:5000/products', newProduct);
setProducts([...products, response.data]); // Add new product to state
setName(''); // Clear input fields
setPrice('');
} catch (error) {
console.error('Error adding product:', error);
}
};
const handleDelete = async (id) => {
try {
await axios.delete(`http://localhost:5000/products/${id}`);
setProducts(products.filter((product) => product.id !== id)); // Remove deleted product from state
} catch (error) {
console.error('Error deleting product:', error);
}
};
if (loading) return <p className="text-center">Loading...</p>;
return (
<div className="p-6">
<h1 className="text-2xl font-bold mb-4">Product List</h1>
{/* Add Product Form */}
<form onSubmit={handleSubmit} className="mb-6">
<div className="mb-4">
<label className="block mb-2">Product Name:</label>
<input
type="text"
value={name}
onChange={(e) => setName(e.target.value)}
className="border rounded px-4 py-2 w-full"
required
/>
</div>
<div className="mb-4">
<label className="block mb-2">Price:</label>
<input
type="number"
value={price}
onChange={(e) => setPrice(e.target.value)}
className="border rounded px-4 py-2 w-full"
required
/>
</div>
<button
type="submit"
className="bg-blue-500 text-white px-4 py-2 rounded hover:bg-blue-600"
>
Add Product
</button>
</form>
{/* Product Table */}
<table className="table-auto w-full border-collapse border border-gray-300">
<thead>
<tr>
<th className="border px-4 py-2">ID</th>
<th className="border px-4 py-2">Name</th>
<th className="border px-4 py-2">Price</th>
<th className="border px-4 py-2">Actions</th>
</tr>
</thead>
<tbody>
{products.map((product) => (
<tr key={product.id}>
<td className="border px-4 py-2">{product.id}</td>
<td className="border px-4 py-2">{product.name}</td>
<td className="border px-4 py-2">${product.price}</td>
<td className="border px-4 py-2">
<button
onClick={() => handleDelete(product.id)}
className="bg-red-500 text-white px-4 py-2 rounded hover:bg-red-600"
>
Delete
</button>
</td>
</tr>
))}
</tbody>
</table>
</div>
);
}