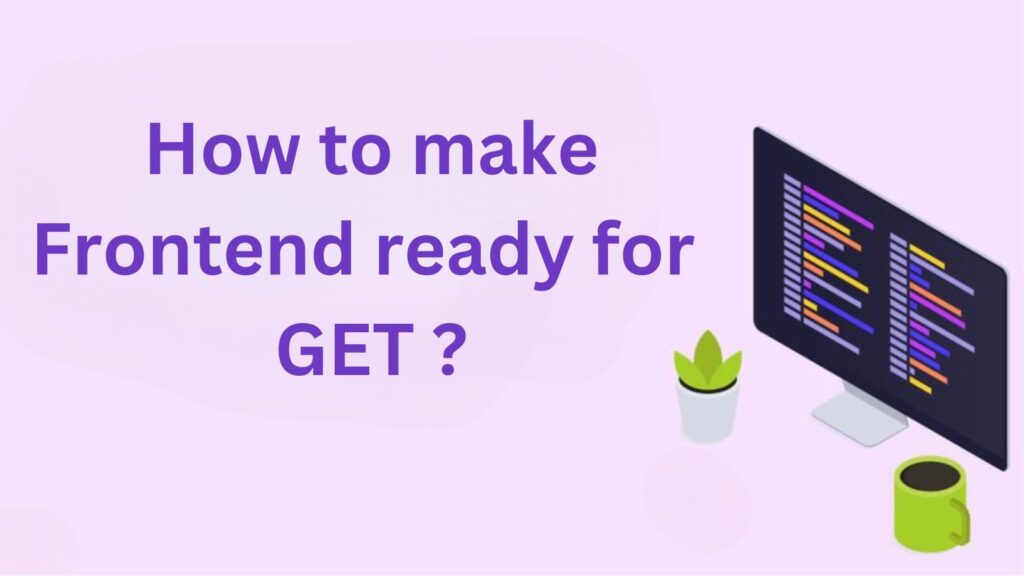
- Fetching data from MySQL and show on website
- Storing data to MySQL from form
How to create ui, connect axios with nextjs, send axios request to send/bring data from Express App?
For Showing: setup the nextjs application —–> create the ui ( for GET – Table/card ) ——> connect axios with nextjs ——-> write axios query to send request to Express URL to bring data ——–> after bringing data, show it on the website
FRONTEND CREATION
- connect axios with nextjs
- write axios query
- connect with backend
STORING DATA INSIDE BACKEND
- now put the Axios POST request to store data
Setup the NextJS Application
Install everything
npx create-next-app@latest my-next-app
npm install axios
Create the UI ( Table ) + Axios GET Query
'use client';
import { useState, useEffect} from 'react';
import axios from 'axios';
export default function Brands() {
const [brands, setBrands] = useState([]);
const [error, setError] = useState(null);
useEffect(() => {
axios.get('http://localhost:8000/api/brands')
.then( (response)=> {
setBrands(response.data);
})
.catch((error)=> {
console.log(error);
setError('Failed to fetch brands. Please try again later.');
});
}, []); // Empty dependency array means this runs once when the component mounts
// Log brands every time they change
useEffect(() => {
console.log('Brands State Updated:', brands);
}, [brands]);
const handleDelete = (id) => {
axios.delete(`http://localhost:8000/api/brands`)
.then(() => {
// Filter out the deleted brand from the state
setBrands(brands.filter(brand => brand.id !== id));
console.log(`Brand with id ${id} deleted`);
})
.catch(err => {
console.error(err);
setError('Failed to delete brand. Please try again later.');
});
};
return (
<div className="min-h-screen flex items-center justify-center bg-gray-100">
<div className="bg-white p-6 rounded-lg shadow-md w-3/4">
<h1 className="text-2xl font-bold mb-4 text-center">All Brands</h1>
<table className="min-w-full border-collapse border border-gray-300">
<thead>
<tr>
<th className="border px-4 py-2">Brand Name</th>
</tr>
</thead>
<tbody>
{console.log('Brands state:', brands)} {/* Log brands before mapping */}
{brands.map((brand, index) => {
return (
<tr key={index}>
<td className="border px-4 py-2 text-center">{brand.brand}
</td>
<td className="border px-4 py-2 text-center">
<button
onClick={() => handleDelete(brand.id)}
className="text-red-500 hover:text-red-700">
Delete
</button>
</td>
</tr>
);
})}
</tbody>
</table>
</div>
</div>
);
}
Run the Next.js Application
Run the development server with npm run dev
.
Send Axios GET Request for a Single Product
'use client';
import { useState, useEffect} from 'react';
import axios from 'axios';
export default function Brands() {
const [product, setProduct] = useState([]);
useEffect(() => {
axios.get(`http://localhost:8000/api/product/:${params.id}`) // params.id will come from URL
.then( (response)=> {
setProduct(response.data);
})
.catch((error)=> {
console.log(error);
setError('Failed to fetch Axios Data. Please try again later.');
});
}, []); // Empty dependency array means this runs once when the component mounts
How to fetch table cell data?
How to fetch images?
How to fetch videos?