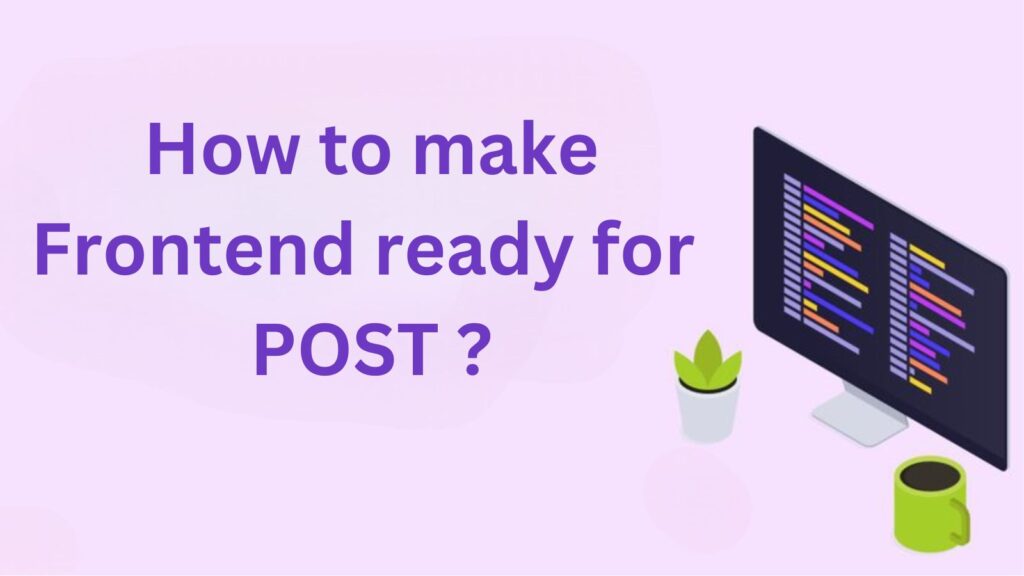
- submitting data from form to store in mysql
- after storing data, show “success” notification on the website
For Submitting: setup the nextjs application —–> create the ui ( for POST – Form ) ——> connect axios with nextjs ——-> write axios query to send request to Express URL to store data ——–> after storing data, show “success” notification on the website
Setup the NextJS Application
Install everything
npx create-next-app@latest my-next-app
npm install axios
Client-Side Example to Send POST Request:
Here’s how you could send this data using a frontend (e.g., React with Axios):
import axios from 'axios';
const productData = {
displayImage: 'image1.jpg',
firstImage: 'image2.jpg',
// Add all other fields...
slug: 'unique-slug',
cardTitle: 'Product Title',
category: 'Category Name',
// Add all other fields...
};
axios.post('/api/add-product', productData)
.then(response => {
console.log('Product added:', response.data);
})
.catch(error => {
console.error('There was an error adding the product:', error);
});
Create the UI ( Form ) + Axios POST Query
import { useEffect, useState } from 'react';
import axios from 'axios';
export default function Products() {
const [products, setProducts] = useState([]);
const [loading, setLoading] = useState(true);
const [name, setName] = useState('');
const [price, setPrice] = useState('');
useEffect(() => {
fetchProducts();
}, []);
const fetchProducts = async () => {
try {
const response = await axios.get('http://localhost:5000/products');
setProducts(response.data);
setLoading(false);
} catch (error) {
console.error('Error fetching products:', error);
}
};
const handleSubmit = async (e) => {
e.preventDefault(); // Prevent page reload
try {
const newProduct = { name, price };
const response = await axios.post('http://localhost:5000/products', newProduct);
setProducts([...products, response.data]); // Add new product to state
setName(''); // Clear input fields
setPrice('');
} catch (error) {
console.error('Error adding product:', error);
}
};
if (loading) return <p className="text-center">Loading...</p>;
return (
<div className="p-6">
<h1 className="text-2xl font-bold mb-4">Product List</h1>
{/* Add Product Form */}
<form onSubmit={handleSubmit} className="mb-6">
<div className="mb-4">
<label className="block mb-2">Product Name:</label>
<input
type="text"
value={name}
onChange={(e) => setName(e.target.value)}
className="border rounded px-4 py-2 w-full"
required
/>
</div>
<div className="mb-4">
<label className="block mb-2">Price:</label>
<input
type="number"
value={price}
onChange={(e) => setPrice(e.target.value)}
className="border rounded px-4 py-2 w-full"
required
/>
</div>
<button
type="submit"
className="bg-blue-500 text-white px-4 py-2 rounded hover:bg-blue-600"
>
Add Product
</button>
</form>
{/* Product Table */}
<table className="table-auto w-full border-collapse border border-gray-300">
<thead>
<tr>
<th className="border px-4 py-2">ID</th>
<th className="border px-4 py-2">Name</th>
<th className="border px-4 py-2">Price</th>
</tr>
</thead>
<tbody>
{products.map((product) => (
<tr key={product.id}>
<td className="border px-4 py-2">{product.id}</td>
<td className="border px-4 py-2">{product.name}</td>
<td className="border px-4 py-2">${product.price}</td>
</tr>
))}
</tbody>
</table>
</div>
);
}
Run the Next.js Application
Run the development server with npm run dev
.
How to implement Multer ?
How to send form fields?
How to send images?
How to send Videos?